Add custom fonts to Matplotlib
This is step by step guide to install and use a new font in Matplotlib. I have tested this procedure on both Linux and OS X machines. Suppose you are running a Jupyter notebook and you want to change the font of a plot. Here is what you need to do!
- First, we need to install our custom font. Keep in mind that Matplotlib expects a font in True Type format (.ttf). For example, if we want to add the Helvetica font, we need to check if we have the font in .ttf format installed on our system otherwise we need to download it and install it.
- Next, we need to update the font cache from the command line with the following command:
sudo fc-cache -fv
- Clear the matplotlib font cache:
rm -fr ~/.cache/matplotlib
- Restart your Jupyter notebook server
That’s it! Now we need to instruct Matplotlib to use our custom font. Suppose that we want to plot a bar chart and change the labels and ticks font. In this case, just for visual purposes, we will use the Comic Sans font.
import pandas as pd
import matplotlib.pyplot as plt
%matplotlib inline
df = pd.DataFrame({'perc': pd.Series([45, 35, 10, 5, 3, 2], index=['A', 'B', 'C','D','E','F'])})
# specify the custom font to use
plt.rcParams['font.family'] = 'sans-serif'
plt.rcParams['font.sans-serif'] = 'Comic Sans MS'
fig, ax = plt.subplots(figsize=(7,4))
df.iloc[::-1].plot(kind='barh', legend = False, ax=ax)
ax.set_xlabel('Percentage',fontsize=15)
ax.set_ylabel('Type',fontsize=15)
Now, let’s try to plot it and see the actual result!
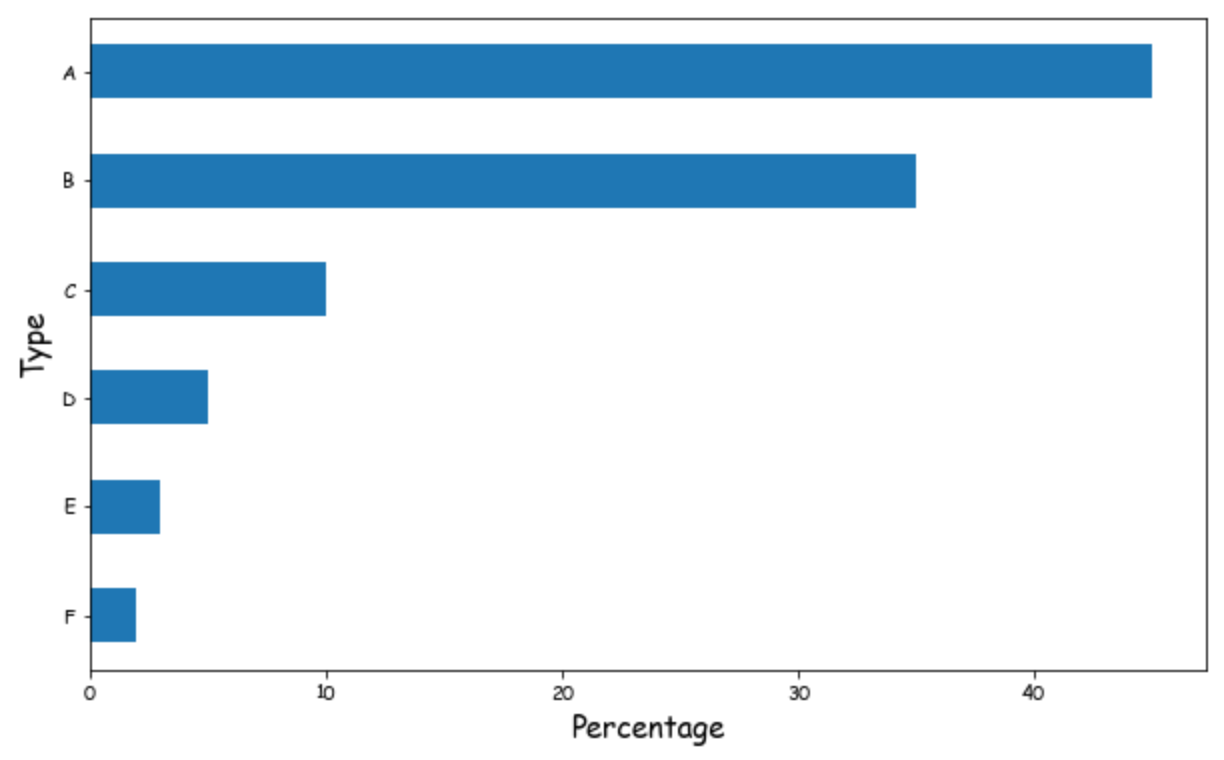
Fig 1. Font changed!
In case something went wrong and you receive an error like this
UserWarning: findfont: Font family [‘sans-serif’] not found. Falling back to DejaVu Sans (prop.get_family(), self.defaultFamily[fontext]))
you need to check whether matplotlib is “seeing” your custom font. In order to do so, we can run in our notebook the following two lines of code
import matplotlib.font_manager
matplotlib.font_manager.findSystemFonts(fontpaths=None, fontext='ttf')
If your font is not in the list, you need to copy your custom font in the system library
- ~/Library/Fonts/ in OS X
- /usr/share/fonts/truetype in Linux
and run again the commands from step 2 to 4.
Add a custom font without installing it
It is possible to use a custom font without installing it in your operating system.
First we download our font (again as an example we will use Comic Sans), and then we save it somewhere on the disk (path/to/font/).
Then we can run the following code to use our choosen font:
from matplotlib import font_manager
font_dirs = ['path/to/font/']
font_files = font_manager.findSystemFonts(fontpaths=font_dirs)
for font_file in font_files:
font_manager.fontManager.addfont(font_file)
# set font
plt.rcParams['font.family'] = 'Comic Sans'
You can see the complete code in this [notebook]